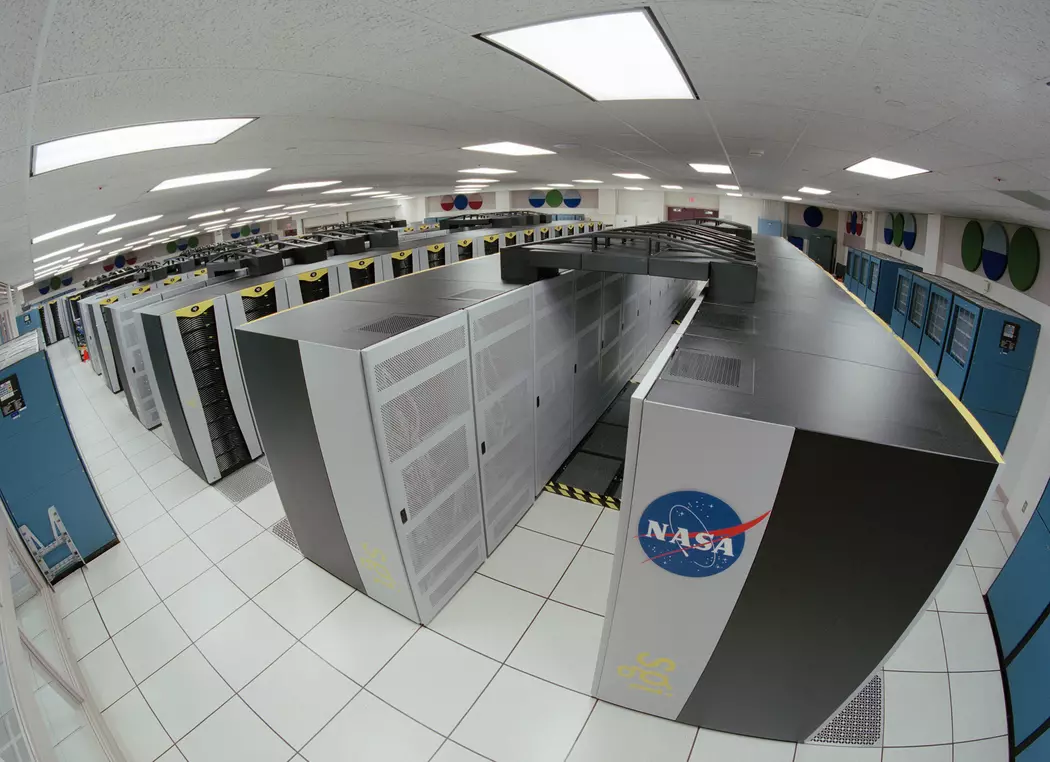
NASA’s been writing mission-critical software for space exploration for decades, and now the organization is turning those guidelines into a coding standard for the software development industry.
The NASA Jet Propulsion Laboratory’s (JPL) Laboratory for Reliable Software recently published a set of code guidelines, “The Power of Ten—Rules for Developing Safety Critical Code.” The paper’s author, JPL lead scientist Gerard J. Holzmann, explained that the mass of existing coding guidelines is inconsistent and full of arbitrary rules, rarely allowing for now-essential tasks such as tool-based compliance checks. Existing guidelines, he said, inundate coders with vague rules, causing code quality of even the most critical applications to suffer.
“Most serious software development projects use coding guidelines,” Holzmann wrote. “These guidelines are meant to state what the ground rules are for the software to be written: how it should be structured and which language features should and should not be used. Curiously, there is little consensus on what a good coding standard is.”
Holzmann laid out 10 strict rules for developing software with code safety in mind. The rules were specifically written with the C language in mind (a language NASA recommended for safety-critical code due to its long history and extensive tool support), though the rules can be generalized for coding in any programming language.
1: Restrict all code to very simple control flow constructs. Do not use GOTO statements, setjmp or longjmp constructs, or direct or indirect recursion.
2: All loops must have a fixed upper bound. It must be trivially possible for a checking tool to statically prove that a preset upper bound on the number of iterations of a loop cannot be exceeded. If the loop-bound cannot be proven statically, the rule is considered violated.
3: Do not use dynamic memory allocation after initialization.
4: No function should be longer than what can be printed on a single sheet of paper (in a standard reference format with one line per statement and one line per declaration.) Typically, this means no more than about 60 lines of code per function.
5: The assertion density of the code should average a minimum of two assertions per function. Assertions must always be side effect-free and should be defined as Boolean tests.
6: Data objects must be declared at the smallest possible level of scope.
7: Each calling function must check non-void function return values, and the validity of parameters must be checked inside each function.
8: Preprocessor use must be limited to the inclusion of header files and simple macro definitions. Token pasting, variable argument lists (ellipses), and recursive macro calls are not allowed.
9: The use of pointers should be restricted. Specifically, no more than one level of dereferencing is allowed. Pointer dereference operations may not be hidden in macro definitions or inside typedef declarations. Function pointers are not permitted.
10: All code must be compiled, from the first day of development, with all compiler warnings enabled at the compiler’s most pedantic setting. All code must compile with these setting without any warnings. All code must be checked daily with at least one—but preferably more than one—state-of-the-art static source code analyzer, and should pass the analyses with zero warnings.
Holzmann included detailed rationales for each of these rules in the paper, but the general gist is that together, the rules guarantee a clear and transparent control flow structure to make it easier to build, test and analyze code along broadly accepted but all-around disjointed standards. JPL has developed automated software for deep space missions such as the Mars Curiosity rover and the Voyager probe, and the laboratory is already using the rules on an experimental basis to write mission-critical software.
Holzmann believed that complying with NASA’s rules, strict as they might be, can lessen the burden on developers and lead to better code clarity, analyzability and safety.
“If the rules seem Draconian at first, bear in mind that they are meant to make it possible to check code where very literally your life may depend on its correctness: code that is used to control the airplane that you fly on, the nuclear power plant a few miles from where you live, or the spacecraft that carries astronauts into orbit,” he wrote.
“The rules act like the seat belt in your car: Initially they are perhaps a little uncomfortable, but after a while their use becomes second-nature, and not using them becomes unimaginable.”
Applying NASA’s coding standards to JavaScript
NASA JPL’s rules for developing safety-critical code are broad enough to generalize to writing code in any programming language, but one developer has already connected the dots to the most popular Web development language out there: JavaScript.
Dutch front-end developer Denis Radin, a.k.a. Pixels Commander, published a blog post breaking down how each of NASA’s 10 rules relates to JavaScript. Check out an abridged rundown of how JavaScript developers can adhere to each rule, and where the benefit lies in doing so.
1: Restrict all code to very simple control flow constructs. “Why [do] NASA guidelines prescribe to avoid simple technique we were studying as early as in school? The reason for that is static code analyzers NASA use to reduce the chance for error,” Radin wrote.
He recommended JavaScript developers use constructs justified by complexity, use code analyzers to reduce defects, monitor codebase metrics, and analyze types with various tools.
2: All loops must have a fixed upper bound. “This makes static analysis more effective and helps to avoid infinite loops,” Radin wrote. “If [the] limit is exceeded, [the] function returns [an] error and this takes [the] system out of failure state. For sure, this is quite valuable for software with 20 years’ uptime!”
3: Do not use dynamic memory allocation after initialization. “Memory leaks often, spoiled JavaScript developers do not have a culture of managing memory, garbage collectors decrease performance when run, and it is hard to tame,” Radin wrote.
Based on the rule, he recommended JavaScript developers manage variables with respect, watch for memory leaks, and switch JavaScript to static memory mode.
4: No function should be longer than what can be printed on a single sheet of paper. “This fits perfectly for JavaScript. Decomposed code is better to understand, verify and maintain,” Radin wrote.
5: The assertion density of the code should average a minimum of two assertions per function. “[The] specialty of assertions is that they execute in runtime…[the] closest practice for JavaScript is a combination of unit tests and runtime checks for program state conformity with generating errors and errors handling,” Radin wrote.
6: Data objects must be declared at the smallest possible level of scope. “This rule [has] simple intentions behind [it]: to keep data in private scope and avoid unauthorized access. Sounds generic, smart and easy to follow,” Radin wrote.
7: Each calling function must check non-void function return values, and the validity of parameters must be checked inside each function. “Authors of [the] guideline assure that this one is the most violated,” Radin wrote. “And this is easy to believe because in its strictest form it means that even built-in functions should be verified. [In] my opinion it makes sense to verify results of third-party libraries being returned to app code, and function-incoming parameters should be verified for existence and type accordance.”
8: Preprocessor use must be limited to the inclusion of header files and simple macro definitions. “Using preprocessors should be limited in any language,” Radin wrote. “They are not needed since [JavaScript has a] standardized, clean and reliable syntax for putting commands into [the] engine.”
9: The use of pointers should be restricted. Specifically, no more than one level of dereferencing is allowed. This is the only rule Radin admitted a JavaScript developer can’t take anything from.
10: All code must be compiled, from the first day of development, with all compiler warnings enabled at the compiler’s most pedantic setting. “We all know it… Do not hoard warnings, do not postpone fixes, keep code clean and [keep the] perfectionist inside you alive,” Radin wrote.
Radin’s full explanations for how JavaScript developers can follow NASA’s ten safety-critical code rules are available in Pixels Commander’s blog post.